Interviews are quite tricky affair, but you can crack most of them if you have prepared with good resource. We have prepared “Important Vue.js Questions With Answers for Interviews” blog so you can answer most important questions in you next Vue interview.
1. What is Vue.js?
Vue.js is a progressive JavaScript framework used for building user interfaces. It focuses on the view layer and provides an intuitive and flexible approach to building web applications. Vue.js is known for its simplicity, scalability, and excellent performance.
2. What are the key features of Vue.js?
Some key features of Vue.js include:
- Reactive data binding: Vue.js provides declarative rendering and automatic updates of the DOM based on the data changes.
- Component-based architecture: Vue.js allows building reusable and modular components.
- Directives: Vue.js offers a set of built-in directives that enable manipulating the DOM easily.
- Vue Router: A powerful routing library that provides seamless navigation between different views in a Vue application.
- Vuex: A state management pattern and library for managing application-level state in Vue.js.
- Vue CLI: A command-line tool for scaffolding Vue.js projects and managing build configurations.
3. Explain the Vue.js component lifecycle hooks.
Vue.js provides several lifecycle hooks that allow you to perform actions at different stages of a component’s lifecycle. Some commonly used hooks are:
beforeCreate
andcreated
: These hooks are called before and after the instance is created, but before data observation and template compilation.beforeMount
andmounted
: These hooks are called before and after the component is inserted into the DOM.beforeUpdate
andupdated
: These hooks are called before and after the component’s data changes, but before and after the DOM is re-rendered.beforeUnmount
andunmounted
: These hooks are called before and after the component is removed from the DOM.
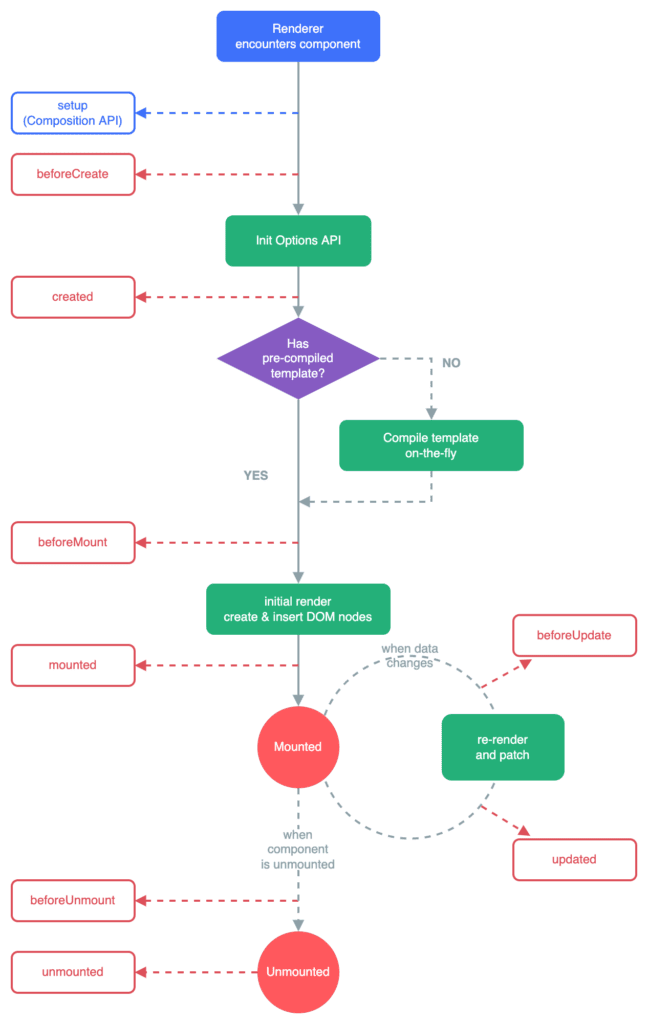
4. How does Vue.js achieve reactivity?
Vue.js achieves reactivity through its reactive data system. It uses a virtual DOM and tracks dependencies between data properties and the associated DOM elements. When a data property changes, Vue.js automatically updates the affected parts of the DOM to reflect the new value. This reactivity is achieved through the use of getters and setters, which allow Vue.js to observe and react to changes in data properties.
5. What is the difference between Vue.js and React?
Vue.js and React are both popular JavaScript frameworks for building user interfaces, but they have some differences:
- Approach: Vue.js takes an opinionated approach and provides a more structured and straightforward development experience, while React is more flexible and allows developers to make their own decisions.
- Learning curve: Vue.js has a gentler learning curve compared to React, making it easier for beginners to get started.
- Size and performance: Vue.js has a smaller bundle size and better performance out-of-the-box compared to React.
- Ecosystem: React has a larger ecosystem and a wide range of community-driven libraries and tools, while Vue.js has a more cohesive and integrated ecosystem.
- Community and adoption: React has a larger community and higher adoption in the industry, while Vue.js has been rapidly growing and gaining popularity.
6. How can you communicate between components in Vue.js?
There are several ways to communicate between components in Vue.js:
- Props: Parent components can pass data to child components using props, which are custom attributes defined in the child component’s template.
- Events: Child components can emit events to notify parent components about changes or trigger actions. Parent components can listen to these events using the
v-on
directive. - Vuex: Vuex is a state management library for Vue.js that allows components to share state in a centralized store. Components can read and modify the shared state using Vuex’s getters and mutations.
- Provide/Inject: The provide/inject mechanism allows a parent component to provide data or methods that can be accessed by its child components, regardless of their nesting level. The parent component uses the
provide
option to expose data or methods, while the child components use theinject
option to access them.
7. What are Vue directives and give examples?
Vue directives are special attributes that provide additional functionality to the DOM elements they are applied to. Some commonly used directives in Vue.js are
v-if
andv-show
: Conditionally render or hide elements based on a condition.v-for
: Render a list of items based on an array or object.v-bind
or:
: Bind an element’s attribute or property to a data property.v-on
or@
: Attach event listeners to elements and bind them to methods.v-model
: Create two-way data binding between form input elements and data properties.v-html
: Render HTML content dynamically.v-slot
or#
: Define slots in components for content insertion.
8. What is computed property in Vue.js?
Computed properties in Vue.js are properties that are derived from the existing data properties and are calculated dynamically. Computed properties are defined as functions in the computed
option of a component and are cached based on their dependencies. They provide a way to perform complex calculations or transformations on data and are reactive, meaning they are automatically updated whenever the dependent data changes.
9. Explain Vue Router and its usage.
Vue Router is the official routing library for Vue.js. It provides seamless navigation between different views or pages in a Vue application. Vue Router allows you to define routes and associate them with corresponding components. It supports dynamic route matching, nested routes, route parameters, and lazy-loading of components. With Vue Router, you can handle navigation, implement authentication, guard routes, and create a Single-Page Application (SPA) experience.
10. What is Vuex and why is it used?
Vuex is a state management pattern and library for Vue.js applications. It serves as a centralized store for managing the application’s state in a predictable and scalable way. Vuex helps to manage shared state between components, making it easy to track changes and maintain consistency across the application. It provides a set of concepts and APIs for defining and manipulating state, mutations for modifying the state, actions for asynchronous operations, getters for accessing computed state properties, and modules for organizing the store into smaller, reusable modules.
11. What is the purpose of the key
attribute in Vue.js and when should it be used?
The key
attribute is used to give a unique identifier
to each rendered element in a loop. When Vue.js re-renders a list of elements, it uses the key
attribute to identify which elements have changed, been added, or been removed. By providing a unique key, Vue.js can optimize the rendering process and improve performance. The key
attribute should be used whenever rendering a list of elements using v-for
, especially when the list items can be dynamically added, removed, or re-ordered.
12. What is the difference between watch
and computed
properties in Vue.js?
The main difference between watch
and computed
properties is their purpose and usage:
watch
: A watch property is used to perform reactive operations in response to changes in one or more data properties. You can define a watch property to watch for changes and trigger a specific function or action when those changes occur.computed
: A computed property is used to derive new data based on existing data properties. Computed properties are cached and only re-evaluated when their dependencies change. Computed properties are used to perform calculations or transformations on data and return the result for easy and efficient usage.
13. What are mixins in Vue.js and why are they useful?
Mixins are a way to share reusable functionality across multiple Vue components. They allow you to define a set of options, such as data, methods, computed properties, and lifecycle hooks, and then apply those options to multiple components. Mixins are useful for reducing code duplication and promoting code reusability. They enable you to extract common functionality into reusable units and apply them to different components, providing a modular and flexible approach to component composition.
14. Explain the concept of Vue’s reactivity system.
Vue’s reactivity system allows you to define reactive data properties that automatically update the associated DOM elements whenever the data changes. When a data property is marked as reactive, Vue.js internally creates a getter and setter for that property. When the property is accessed or modified, Vue.js detects the dependency and triggers the necessary updates in the DOM. This reactivity system simplifies the process of managing and synchronizing the data and the view, enabling efficient rendering and a smooth user experience.
15. How can you optimize the performance of a Vue.js application?
Some techniques to optimize the performance of a Vue.js application include:
- Implementing lazy-loading or code splitting to load components and resources only when needed.
- Using Vue’s production mode for better performance and smaller bundle size.
- Applying appropriate data caching strategies to minimize unnecessary re-rendering.
- Using
key
attributes when rendering lists to optimize element reusability. - Minimizing the use of watchers and using computed properties instead when possible.
- Utilizing asynchronous rendering with
v-once
orSuspense
components to prioritize critical content loading. - Employing code profiling and performance monitoring tools to identify and address bottlenecks.
16. What is the purpose of the v-model
directive in Vue.js?
The v-model
directive in Vue.js is a two-way data binding directive. It is commonly used with form input elements, such as <input>
, <textarea>
, and <select>
. The v-model
directive allows you to bind form inputs to a data property in Vue’s component instance. It automatically synchronizes the input value with the data property, so any changes made to the input are reflected in the data property, and vice versa.
17. How can you handle user input validation in Vue.js?
- Vue.js provides various mechanisms for handling user input validation, including:
- Using built-in HTML5 form validation attributes like
required
,min
,max
, etc. - Leveraging Vue’s computed properties or watchers to perform custom validation logic based on the data entered by the user.
- Utilizing third-party validation libraries, such as Vuelidate or VeeValidate, which offer more advanced validation features and error handling capabilities.
- Using built-in HTML5 form validation attributes like
18. Explain the concept of slots in Vue.js.
Slots are a powerful feature in Vue.js that allows you to define placeholders in component templates where content can be injected from the parent component. They provide a way to pass content, such as text, HTML, or other components, into a component’s template. Slots enable component reusability and customization by allowing developers to define flexible layouts and provide dynamic content based on specific use cases.
19. How can you handle asynchronous operations in Vue.js?
Vue.js provides multiple options for handling asynchronous operations, such as:
- Using
async/await
syntax within methods to make asynchronous calls and wait for the results before proceeding. - Utilizing the
created
ormounted
lifecycle hooks to initiate asynchronous operations. - Employing third-party libraries, such as Axios or Vue Resource, for making AJAX requests and handling responses.
- Incorporating Vuex actions for managing asynchronous state changes in a centralized store.
20. What is the purpose of Vue’s single-file components (SFCs)?
Single-file components (SFCs) are a Vue.js feature that allows you to define components in a single file, combining the template, JavaScript, and styles together. SFCs improve component organization, maintainability, and reusability by providing a clear separation of concerns within a single file. They enable better collaboration between developers and support enhanced tooling, such as linting, syntax highlighting, and component documentation.
21. Explain the concept of scoped styles in Vue.js.
Scoped styles in Vue.js are a feature that allows you to define component-specific styles that are scoped to that particular component only. By using the scoped
attribute in the <style>
tag of a component, Vue.js automatically adds a unique identifier to each CSS class or selector in that component’s styles. This ensures that the styles defined in one component do not affect other components in the application, promoting encapsulation and preventing unintended style conflicts.
22. How can you handle route-based code splitting in Vue.js?
Route-based code splitting allows you to dynamically load different parts of your Vue.js application based on the routes the user navigates to. Vue Router provides a built-in mechanism for route-based code splitting using dynamic import statements. By defining the routes of your application with corresponding component imports, Vue Router automatically loads the required components when the corresponding routes are accessed, improving initial page load performance.
23. What are mixins and when should you use them in Vue.js?
Mixins in Vue.js allow you to reuse component options across multiple components. They are similar to traits or mixins in
other programming languages. Mixins enable you to encapsulate reusable logic, such as computed properties, methods, or lifecycle hooks, and apply them to multiple components. You can define a mixin as an object with the desired options, and then use the mixins
property in a component to include the mixin’s functionality. Mixins are useful when you need to share common functionality across different components without the need for a full inheritance hierarchy.
24. How can you handle errors and perform error handling in Vue.js?
Vue.js provides various ways to handle errors and perform error handling:
- Using
try/catch
blocks to catch and handle errors within methods or computed properties. - Utilizing the
errorCaptured
lifecycle hook at the component level to capture and handle errors that occur within child components. - Employing global error handling through the
Vue.config.errorHandler
property to handle uncaught errors across the entire application. - Incorporating third-party error tracking services, such as Sentry or Bugsnag, to collect and monitor errors in a production environment.
25. What is the purpose of Vue Devtools and how can you use it?
Vue Devtools is a browser extension that provides a set of development tools specifically designed for debugging and inspecting Vue.js applications. It allows you to inspect the component hierarchy, view and modify component data, monitor state changes, track performance, and debug Vuex stores. Vue Devtools greatly enhances the development experience by providing real-time insights into your Vue.js application’s structure, behavior, and performance. You can install the Vue Devtools extension in your preferred web browser and use it to debug Vue.js applications.
26. How can you perform server-side rendering (SSR) with Vue.js?
Server-side rendering (SSR) with Vue.js involves rendering your Vue components on the server and sending the pre-rendered HTML to the client. SSR improves initial page load performance, enhances search engine optimization (SEO), and provides a better user experience. To implement SSR with Vue.js, you can use frameworks like Nuxt.js, which is built on top of Vue.js and provides built-in server-side rendering capabilities. Nuxt.js simplifies the setup and configuration required for SSR and provides server-side rendering out of the box.
More Blog on Vue.js : https://coderboi.com/category/vue/
Vue.js Official Documentation: https://vuejs.org/guide/