In Vue 2, if you’re getting a warning about mutating props directly (commonly known as the “Vue warn” message), it’s because you’re attempting to modify a prop directly within a child component. Vue follows a unidirectional data flow, where props are meant to be passed from parent components to child components and should not be directly modified by the child components.
Vue.js warning – Avoid mutating a prop directly – How to fix?
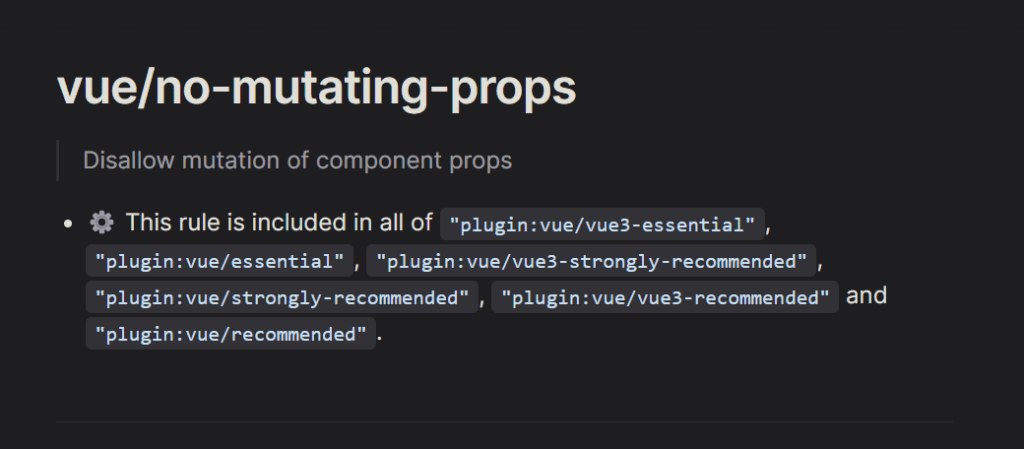
Here’s what the warning might look like:
[Vue warn]: Avoid mutating a prop directly since the value will be overwritten whenever the parent component re-renders.
To resolve this issue, you should follow these steps:
1. Parent Component (Passing Props): Make sure you’re passing the props to the child component in the parent component’s template. Props are meant to be a way for parent components to provide data to their children.
<template>
<child-component :propName="dataFromParent" />
</template>
<script>
export default {
data() {
return {
dataFromParent: "Some data"
};
}
};
</script>
2. Child Component (Receiving Props): In the child component, define the prop and use it as needed. However, do not directly modify the prop.
<template>
<div>
<p>{{ propName }}</p>
</div>
</template>
<script>
export default {
props: {
propName: String
}
};
</script>
3. Mutations in Child Component: If you need to modify the data from the prop, consider creating a local data property in the child component and then performing the modifications there. This way, you won’t be modifying the original prop directly.
<template>
<div>
<p>{{ modifiedData }}</p>
</div>
</template>
<script>
export default {
props: {
propName: String
},
data() {
return {
modifiedData: this.propName
};
},
methods: {
modifyData() {
this.modifiedData = "Modified value";
}
}
};
</script>
By following these steps, you’ll avoid the Vue warning and adhere to Vue’s recommended approach for handling props and data flow within components.
Online Example/Demo : https://codesandbox.io/s/how-to-resolve-avoid-mutating-a-prop-directly-warning-8tmdx5?file=/src/App.vue
Why Vue.js does not recommend modifying props in Child Component.
In Vue, props are used to pass data from parent components to child components. This mechanism ensures that child components remain isolated and don’t directly modify the data they receive from parents. This separation of concerns follows the principle of unidirectional data flow, which helps in maintaining a clear and predictable state management.
When you define a prop in a child component, it becomes a reactive property that is bound to the data provided by the parent component. This means that changes in the parent’s data will automatically propagate down to the child component. However, Vue enforces immutability for props because if a child component were allowed to modify the prop, it could lead to unexpected behavior and make the application harder to reason about.
The warning you encounter, often referred to as the “Vue warn,” is a safeguard put in place by Vue to alert you when you attempt to modify a prop directly within a child component. The warning is meant to prevent accidental prop mutation and to encourage you to follow the unidirectional data flow pattern.
By modifying a prop directly in a child component, you would be breaking the isolation between parent and child components, potentially causing:
- Unpredictable Behavior: Since changes to the prop directly made in the child would be overwritten when the parent component re-renders, the child component might exhibit unexpected behavior.
- Debugging Challenges: Debugging becomes harder when changes can occur from multiple sources (parent and child) without a clear trail of changes.
- Code Maintenance Complexity: As the application grows, it becomes challenging to track where changes to props are coming from, leading to harder-to-maintain code.
Vue’s recommended approach is to treat props as read-only data in child components and manage modifications within the child component’s local state (using data properties) or through the use of events and custom events. This maintains the separation of concerns and helps create more predictable and maintainable code.
Vuejs official Documentation: https://eslint.vuejs.org/rules/no-mutating-props.html
More posts on Vuejs: https://coderboi.com/category/vue/