DataTables is a powerful and flexible jQuery plugin that enhances HTML tables by providing advanced features for sorting, searching, pagination, and data manipulation. It allows you to transform a basic HTML table into a dynamic and interactive data grid with minimal effort. In this blog we will learn “How to Use DataTables with Vue 3 and Bootstrap”.
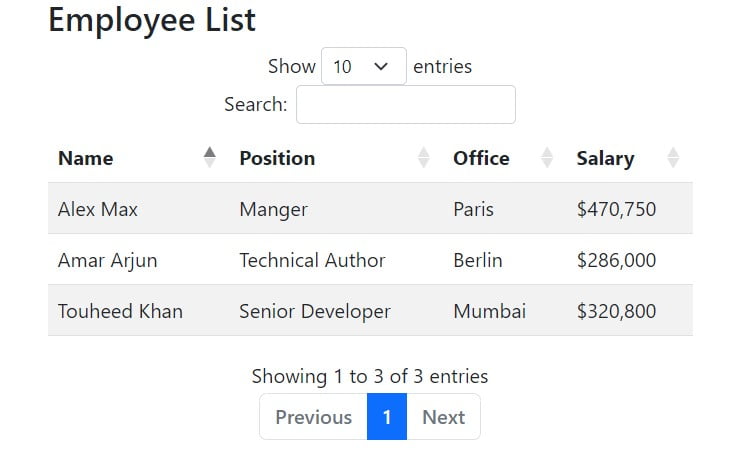
1. Install Dependencies
We need to install 3 dependencies for it: bootstrap, datatables.net-bs5, datatables.net-vue3.
Run below commands to install dependencies:
npm install bootstrap@5.1.3
datatables.net-bs5@1.13.1
datatables.net-vue3@2.1.0
2. Import Dependencies into component
import DataTable from 'datatables.net-vue3';
import DataTablesCore from 'datatables.net-bs5';
DataTable.use(DataTablesCore);
3. Store Data into local variable
You can fetch data using API call or from any source, for our example we are using hard coded data.
const data = [
{
id: '1',
name: 'Touheed Khan',
position: 'Senior Developer',
salary: '$320,800',
office: 'Mumbai',
},
{
id: '2',
name: 'Alex Max',
position: 'Manger',
salary: '$470,750',
office: 'Paris',
},
{
id: '3',
name: 'Amar Arjun',
position: 'Technical Author',
salary: '$286,000',
office: 'Berlin',
},
];
4. Define columns table
Define columns to with correct key name as present in data object. Order of column should be same as order in which you want to display columns in DataTable.
const columns = [
{ data: 'name' },
{ data: 'position' },
{ data: 'office' },
{ data: 'salary' },
];
5. Write template code for DataTable
<DataTable
:columns="columns"
:data="data"
class="table table-hover table-striped"
width="100%"
>
<thead>
<tr>
<th>Name</th>
<th>Position</th>
<th>Office</th>
<th>Salary</th>
</tr>
</thead>
</DataTable>
6. Lastly import DataTable CSS
<style>
@import 'bootstrap';
@import 'datatables.net-bs5';
</style>
7. Run your code and see the magic!
Complete Code
<script setup lang="ts">
import DataTable from 'datatables.net-vue3';
import DataTablesCore from 'datatables.net-bs5';
DataTable.use(DataTablesCore);
const data = [
{
id: '1',
name: 'Touheed Khan',
position: 'Senior Developer',
salary: '$320,800',
office: 'Mumbai',
},
{
id: '2',
name: 'Alex Max',
position: 'Manger',
salary: '$470,750',
office: 'Paris',
},
{
id: '3',
name: 'Amar Arjun',
position: 'Technical Author',
salary: '$286,000',
office: 'Berlin',
},
];
const columns = [
{ data: 'name' },
{ data: 'position' },
{ data: 'office' },
{ data: 'salary' },
];
</script>
<template>
<div class="container">
<h2>Employee List</h2>
<DataTable
:columns="columns"
:data="data"
class="table table-hover table-striped"
width="100%"
>
<thead>
<tr>
<th>Name</th>
<th>Position</th>
<th>Office</th>
<th>Salary</th>
</tr>
</thead>
</DataTable>
</div>
</template>
<style>
@import 'bootstrap';
@import 'datatables.net-bs5';
</style>
Online Demo and StackBlitz Code : Full Code and Demo
More Vue Blogs: https://coderboi.com/category/vue/