In my career I have given tons of interviews to find suitable job for me. From my experience I have curated list of most important Spring Boot questions to crack interview easily.
- What is Spring Boot?
- Answer: Spring Boot is an open-source framework that simplifies the development of Java applications. It provides a set of opinionated defaults, auto-configuration, and production-ready features to accelerate application development.
- How does Spring Boot differ from the traditional Spring framework?
- Answer: Spring Boot builds on top of the traditional Spring framework by providing convention-over-configuration and auto-configuration. It eliminates the need for manual configuration, reduces boilerplate code, and simplifies dependency management. Spring Boot also includes an embedded server, making it easy to create standalone applications.
- What is auto-configuration in Spring Boot?
- Answer: Auto-configuration is a key feature of Spring Boot. It automatically configures the application based on the classpath dependencies, eliminating the need for explicit configuration. Spring Boot scans the classpath for specific libraries or annotations and configures the application accordingly.
- How can you configure a datasource in Spring Boot?
- Answer: In Spring Boot, you can configure a datasource by providing the necessary properties in the
application.properties
orapplication.yml
file. For example, you can specify the URL, username, and password for the database connection. Spring Boot will automatically configure a datasource bean based on these properties.
- Answer: In Spring Boot, you can configure a datasource by providing the necessary properties in the
- What is the purpose of the
@SpringBootApplication
annotation?- Answer: The
@SpringBootApplication
annotation is a convenience annotation that combines three commonly used annotations:@Configuration
,@EnableAutoConfiguration
, and@ComponentScan
. It indicates that a class is the starting point of a Spring Boot application, enabling auto-configuration and component scanning.
- Answer: The
- How can you handle exceptions in Spring Boot?
- Answer: Spring Boot provides various mechanisms to handle exceptions. You can use the
@ControllerAdvice
annotation to create a global exception handler that handles exceptions across the application. Additionally, you can use the@ExceptionHandler
annotation at the controller level to handle specific exceptions.
- Answer: Spring Boot provides various mechanisms to handle exceptions. You can use the
- Explain the concept of profiles in Spring Boot.
- Answer: Profiles in Spring Boot allow you to define different configurations for different environments. By using profiles, you can customize the application’s behavior based on the environment it is running in, such as development, testing, or production. Profiles can be specified in the
application.properties
orapplication.yml
file using thespring.profiles.active
property.
- Answer: Profiles in Spring Boot allow you to define different configurations for different environments. By using profiles, you can customize the application’s behavior based on the environment it is running in, such as development, testing, or production. Profiles can be specified in the
- How does Spring Boot support externalized configuration?
- Answer: Spring Boot supports externalized configuration by allowing properties to be defined outside the application code, in properties files, YAML files, environment variables, or command-line arguments. This makes it easy to modify the application’s behavior without changing the compiled code.
- Explain the Spring Boot Actuator.
- Answer: Spring Boot Actuator is a feature that provides endpoints to monitor and manage a Spring Boot application. It includes endpoints for health checks, metrics, info, logging, and more. Actuator endpoints can be accessed via HTTP or JMX and provide useful insights into the application’s runtime behavior.
- How can you deploy a Spring Boot application?
- Answer: Spring Boot applications can be deployed in various ways. You can create a standalone JAR file and run it using the
java -jar
command. Spring Boot also supports deploying applications to containers like Tomcat or Jetty. Additionally, you can package the application as a Docker image or deploy it to cloud platforms like AWS or Azure.
- Answer: Spring Boot applications can be deployed in various ways. You can create a standalone JAR file and run it using the
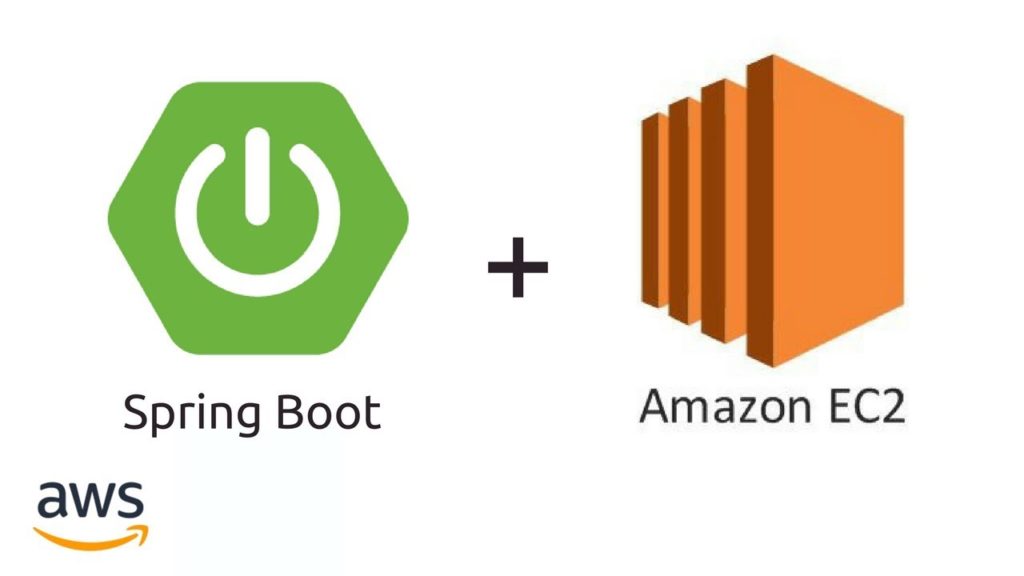
- What is the purpose of the
@RestController
annotation in Spring Boot?
- Answer: The
@RestController
annotation is used to mark a class as a RESTful controller. It combines the@Controller
and@ResponseBody
annotations, indicating that the class methods will handle incoming HTTP requests and return the response directly in the desired format (JSON, XML, etc.).
- How can you handle form validation in Spring Boot?
- Answer: Spring Boot integrates with the Bean Validation API, allowing you to perform form validation easily. You can annotate form fields in your DTO or entity classes with validation annotations like
@NotNull
,@Size
, etc. Spring Boot will automatically validate the data and return validation errors if any.
- What is the purpose of Spring Boot Starter dependencies?
- Answer: Spring Boot Starter dependencies are a set of opinionated dependencies that provide commonly used configurations and libraries for specific functionalities. By including a Starter dependency in your project, you get all the necessary dependencies and auto-configuration required for that particular feature, making it easier to start working on it.
- How can you implement caching in Spring Boot?
- Answer: Spring Boot provides built-in support for caching through integration with popular caching libraries like Ehcache, Caffeine, and Redis. You can enable caching by adding the
@EnableCaching
annotation to your configuration class and use the@Cacheable
and@CacheEvict
annotations to cache and evict data respectively.
- What is the difference between
@Component
,@Service
,@Repository
, and@Controller
annotations in Spring Boot?
- Answer: The
@Component
annotation is a generic stereotype annotation used for any Spring-managed component.@Service
is used to annotate classes that perform business logic or service operations.@Repository
is used to annotate classes that interact with the database.@Controller
is used for classes that handle HTTP requests in a web application.
- How can you secure REST APIs in Spring Boot using OAuth or JWT?
- Answer: Spring Security provides comprehensive support for securing REST APIs. You can use OAuth 2.0 or JSON Web Tokens (JWT) for authentication and authorization. Spring Security offers various configuration options and components to handle token-based authentication and authorization, allowing you to secure your APIs effectively.
- How can you handle cross-origin resource sharing (CORS) in Spring Boot?
- Answer: Spring Boot provides easy configuration to enable CORS support. You can use the
@CrossOrigin
annotation at the controller level or configure CORS globally using theWebMvcConfigurer
interface. This allows you to specify the allowed origins, methods, headers, and other CORS-related settings.
- What is Spring Boot’s Actuator and what are some of its endpoints?
- Answer: Spring Boot Actuator is a feature that provides insight into the application’s internal workings at runtime. It exposes various management endpoints, such as
/health
for health checks,/info
for application information,/metrics
for metrics data,/loggers
for logging configuration, and more. Actuator endpoints help monitor and manage the application in production environments.
- How can you write unit tests for Spring Boot applications?
- Answer: Spring Boot provides testing support through the
spring-boot-starter-test
dependency. You can write unit tests using frameworks like JUnit or TestNG and utilize Spring Boot’s@SpringBootTest
annotation to load the Spring context. You can also use@MockBean
to mock dependencies and@AutoConfigureMockMvc
for testing MVC endpoints.
Read more Java blog from here : https://coderboi.com/category/spring-boot/
Spring Boot official documentation: https://docs.spring.io/spring-boot/docs/current/reference/html/documentation.html