Nowadays interviewers are more focused on programming skills of candidate than his theoretical knowledge in Java. When I was giving interview few weeks back almost in every interview I had to write programs to showcase my programming and logical ability. I have listed Most Asked Programs In Java Interview based on my latest experience.
Print duplicate characters from given string
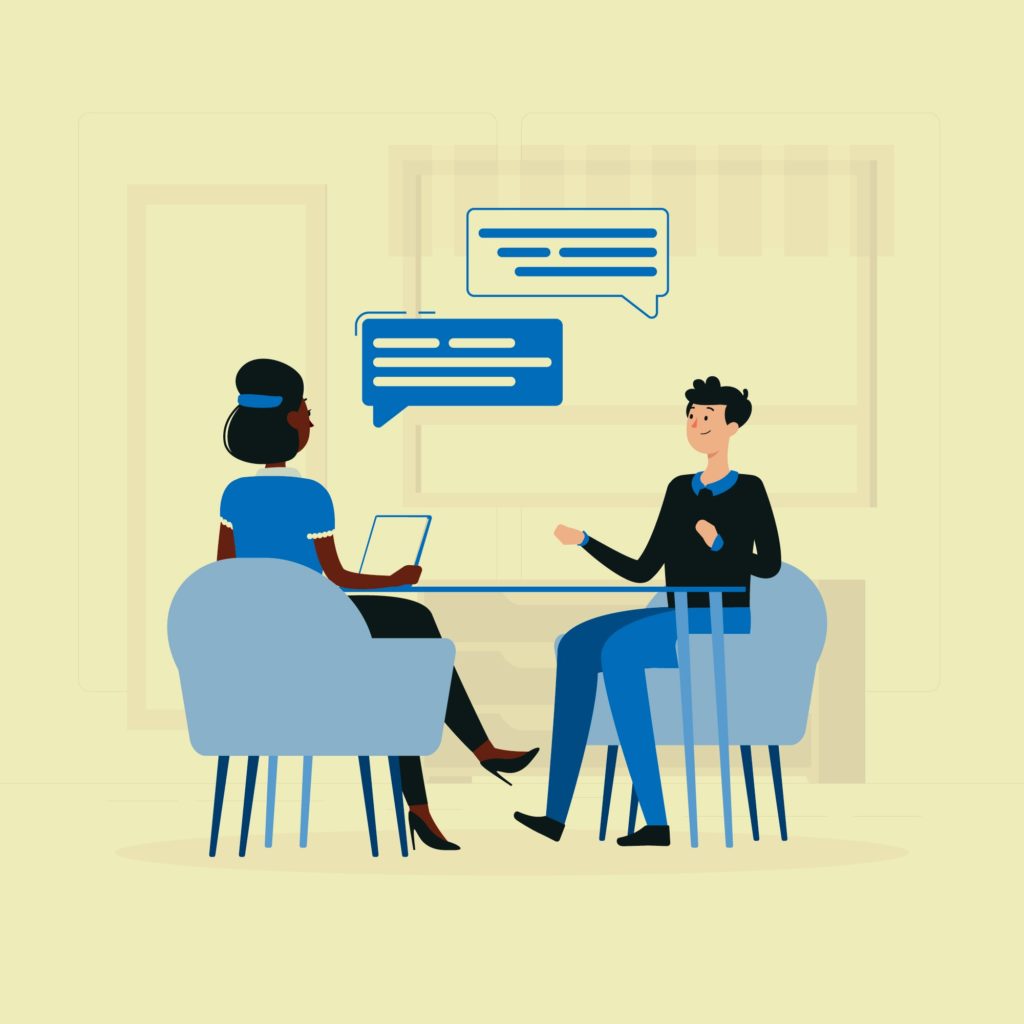
Write a java program to print duplicate characters from given string.
To find the duplicate characters from the string. We iterate over string and count the occurrence of each character. If count is greater than 1, we consider that character as duplicate.
public class DuplicateString {
public static void main(String[] args) {
String str = "Hellooo";
String duplicate = "";
for (int i = 0; i < str.length(); i++) {
for (int j = i +1; j < str.length(); j++) {
if (str.charAt(i) == str.charAt(j) && !duplicate.contains(str.charAt(j) + "")) {
duplicate = duplicate +str.charAt(i);
}
}
}
System.out.println("Duplicate Words: " + duplicate);
}
}
Output
Duplicate Words: lo
Print duplicate words from string
Write a java program to print duplicate words from string
I have used List to store duplicate words and HashSet cause it holds only unique entries it is best option to store duplicate unique words.
import java.util.*;
public class DuplicateWords {
public static void main(String args[]) {
String sentence = "learn Java with coderboi.com Visit coderboi.com to read blogs on Java Spring Boot and other technologies";
sentence = sentence.toLowerCase();
String[] words = sentence.split(" ");
List<String> duplicateWords = new ArrayList<>();
HashSet<String> uniqueWords = new HashSet<>();
for(String str : words)
{
if (!uniqueWords.add(str))
duplicateWords.add(str);
}
System.out.println("Duplicate words are: " + duplicateWords);
}
}
Output
Duplicate words are: [coderboi.com, java]
Check if given string is palindrome or not?
public class Palindrome {
public static void main(String[] args) {
String str = "malayalam";
String result = str.equals( new StringBuilder(str).reverse().toString()) ? "Yes" : "No";
System.out.println( str + " is palindrome: " + result);
}
}
Output
malayalam is palindrome: Yes
Reverse given string
public class ReverseString {
public static void main(String[] args) {
String str = "coderboi.com";
StringBuilder newStr = new StringBuilder();
newStr.append(str);
newStr = newStr.reverse();
System.out.println("Reversed string is : " + newStr);
}
}
Output
Reversed string is : moc.iobredoc
Reverse string without using inbuild function.
public class ReverseStringWithoutFunc {
public static void main(String[] args) {
String str = "coderboi.com";
String[] token = str.split("");
for(int i=token.length-1; i>=0; i--)
{
System.out.print(token[i] + "");
}
}
}
Output
Reversed string is : moc.iobredoc
Swap two number without using third variable.
public class SwapNumber {
public static void main(String[] args) {
int x = 10;
int y = 5;
System.out.println("Before Swap: \nx = "+x+"\ny = "+y);
x = x + y;
y = x - y;
x = x - y;
System.out.println("After Swap: \nx = "+x+"\ny = "+y);
}
}
Output
Before Swap:
x = 10
y = 5
After Swap:
x = 5
y = 10
Program to find if number is prime or not?
public class PrimeNumber {
public static void main(String[] args) {
int temp, num = 11;
boolean isPrime = true;
for (int i = 2; i<= num/2; i++) {
temp = num%i;
if (temp == 0) {
isPrime = false;
break;
}
}
if(isPrime) {
System.out.println(num + " is a prime number");
} else {
System.out.println(num + " is not a prime number");
}
}
}
Output
11 is a prime number
More Blogs on Java: https://coderboi.com/category/java/
Official Java Documentation: https://docs.oracle.com/en/java/